Displaying an array of images in a Vue.js application is a common task, especially when you are working on projects that involve handling and showcasing multiple images. Vue.js, with its simplicity and flexibility, makes this process relatively straightforward. In this comprehensive guide, we will explore how to display an array of images using Vue.js and understand the code snippet provided
Displaying an Array of Images in Vue.js: A Comprehensive Guide
To get started, we have a basic Vue.js template in our HTML file. It’s important to ensure you have Vue.js included in your project, either by linking to a CDN or using a package manager like npm or yarn to install it.
<div id="app">
<h3> Vue Js Display Image from Array</h3>
<div class="image-gallery">
<div v-for="imageObject in imageObjects" :key="imageObject.src" class="image-item">
<img :src="imageObject.src" :alt="imageObject.title">
<p>{{ imageObject.title }}</p>
</div>
</div>
</div>
In this code, we define a Vue.js app with an array of imageObjects
that contains information about each image, including its title and source URL. We then use the v-for
directive to loop through this array and generate a list of image items. The :key
attribute is set to the image source to help Vue.js efficiently update and re-render the DOM when changes occur.
For each image item, we display the image using the img
tag, binding the src
attribute to the src
property of the current imageObject
. We also set the alt
attribute to the title
property to provide alternative text for the image,
Now, let’s take a look at the JavaScript part of our code:
Vue js loop through an array of images json
<script type="module">
const app = Vue.createApp({
data() {
return {
imageObjects: [
{
title: 'image1.jpg',
src: 'https://www.sarkarinaukriexams.com/images/import/sne4446407201.png',
},
{
title: 'image2.jpg',
src: 'https://www.sarkarinaukriexams.com/images/import/sne86811832.png',
},
{
src: 'https://www.sarkarinaukriexams.com/images/import/sne1586776004.png',
title: 'Image 3 Title',
},
// Add more objects with image URLs and titles here
],
}
},
});
app.mount("#app");
</script>
In this JavaScript code, we create a Vue.js app using Vue.createApp
. Inside the data
function, we define the imageObjects
array, where each object represents an image with a title
and src
property. You can easily expand this array by adding more objects with image URLs and titles.
Output of Vue js loop through an array of images json
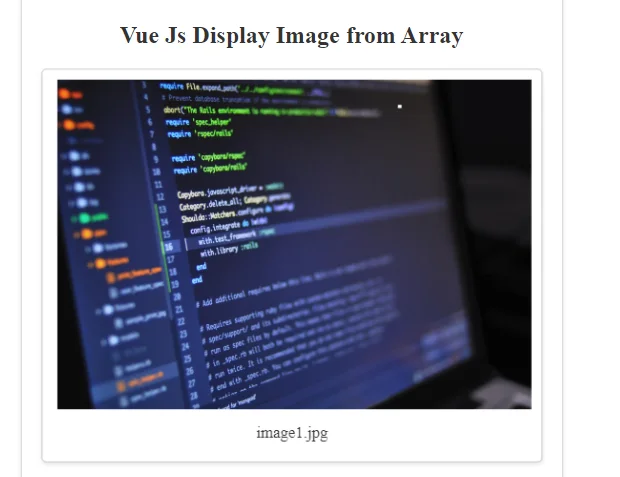
In conclusion, Vue.js’s simplicity and flexibility make it a powerful choice for creating dynamic and visually appealing image galleries. This guide provides you with a solid foundation to display an array of images in your Vue.js application.